Problem Statement
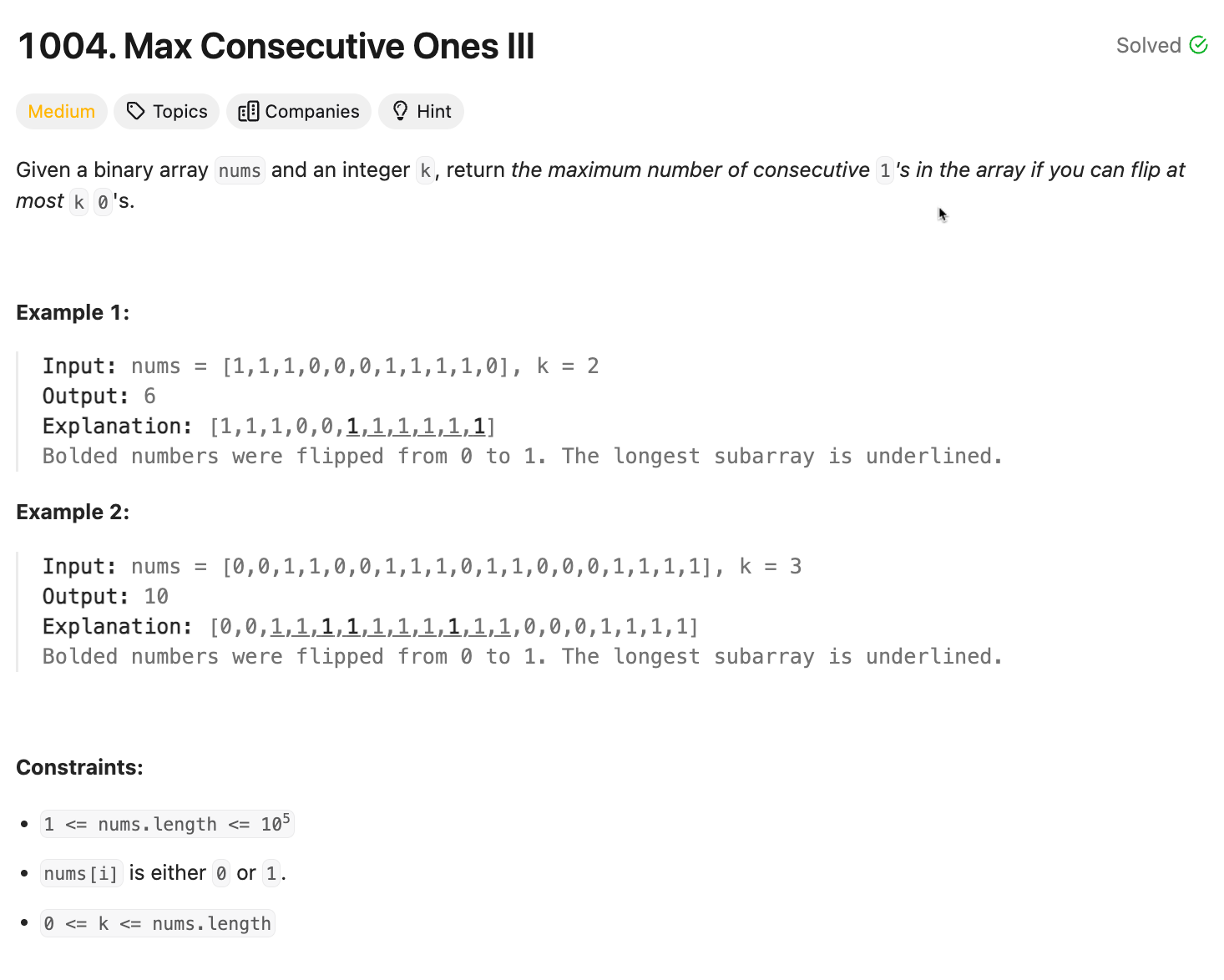
My Solution
class Solution:
def longestOnes(self, nums: List[int], k: int) -> int:
N = len(nums)
res = 0
curr = 0
start = 0
for end in range(N):
if nums[end] == 0:
k -= 1
if k < 0:
while start <= end and k < 0:
if nums[start] == 0:
k += 1
curr -= 1
start += 1
if k >= 0:
curr += 1
res = max(res, curr)
return res
Editorial Solution
class Solution:
def longestOnes(self, nums: List[int], k: int) -> int:
left = 0
for right in range(len(nums)):
# If we included a zero in the window we reduce the value of k.
# Since k is the maximum zeros allowed in a window.
k -= 1 - nums[right]
# A negative k denotes we have consumed all allowed flips and window has
# more than allowed zeros, thus increment left pointer by 1 to keep the window size same.
if k < 0:
# If the left element to be thrown out is zero we increase k.
k += 1 - nums[left]
left += 1
return right - left + 1