Problem Statement
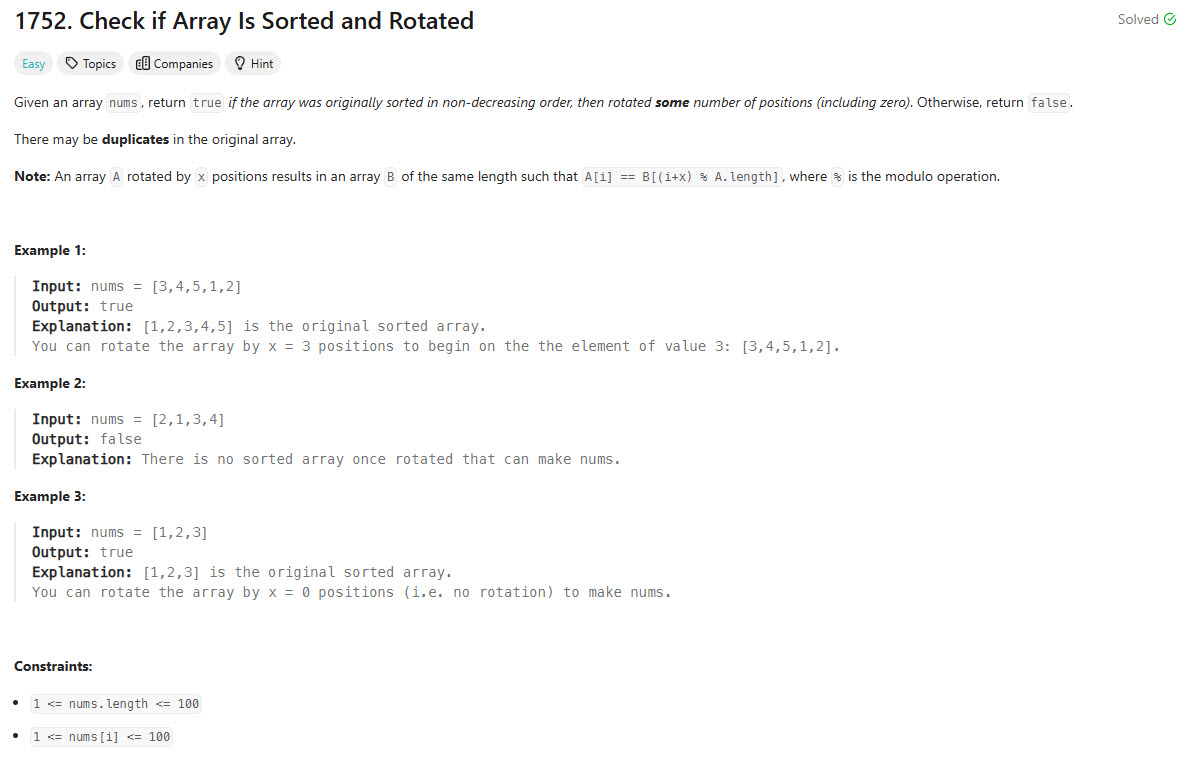
Brute Force [Accepted]
class Solution:
def check(self, nums: List[int]) -> bool:
sorted_nums = sorted(nums)
N = len(nums)
min_val = sorted_nums[0]
start_indices = [index for index, val in enumerate(nums) if val == min_val]
res = False
for start in start_indices:
i = 0
for _ in range(N):
if nums[start] != sorted_nums[i]:
break
i += 1
start = (start + 1) % N
else:
res = True
break
return res
Editorial
Approach 2: Compare with sorted array
class Solution:
def check(self, nums: List[int]) -> bool:
size = len(nums)
# Create a sorted copy of the list
sorted_nums = sorted(nums)
# Compare the original list with the sorted list, considering all possible rotations
for rotation_offset in range(size):
is_match = True
for index in range(size):
if nums[(rotation_offset + index) % size] != sorted_nums[index]:
is_match = False
break
if is_match:
return True
return False