Problem Statement
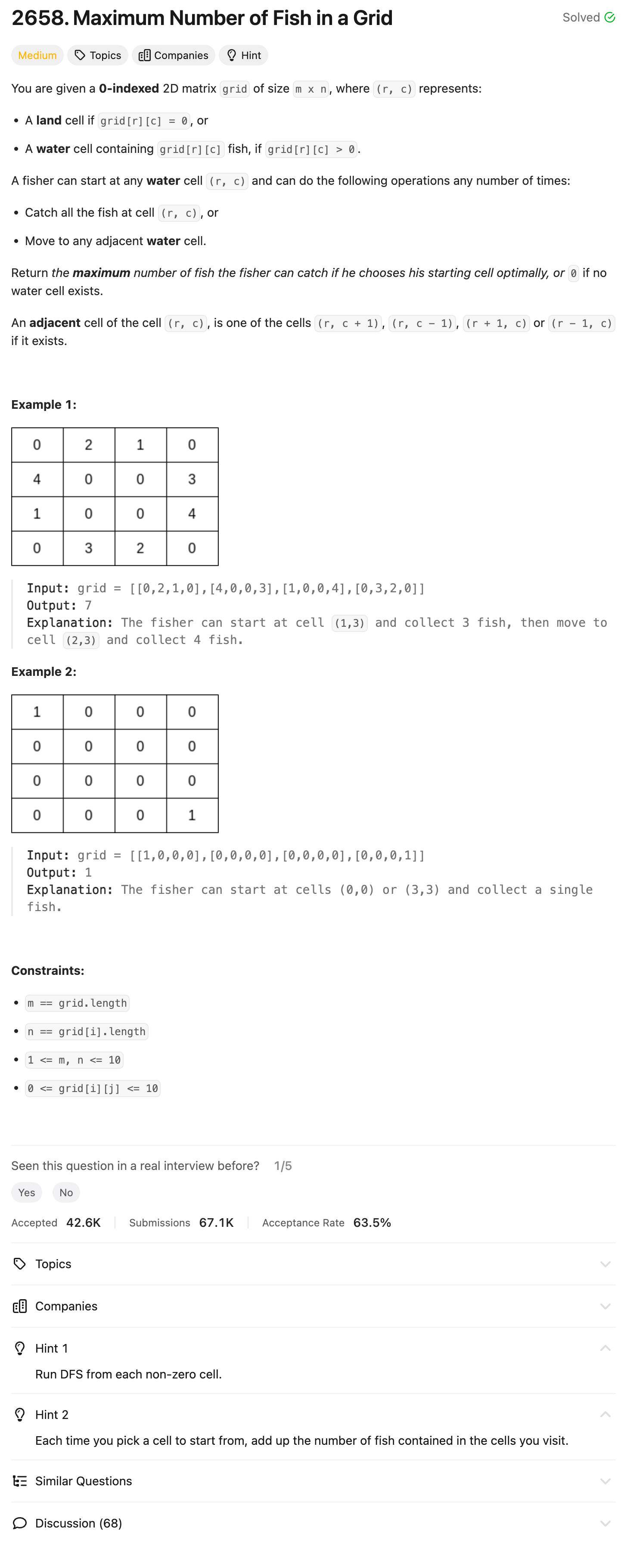
My Solution
class Solution:
def mergeArrays(self, nums1: List[List[int]], nums2: List[List[int]]) -> List[List[int]]:
hash_map = defaultdict(list)
for idx, val in nums1:
hash_map[idx].append(val)
for idx, val in nums2:
hash_map[idx].append(val)
res = []
for idx, vals in hash_map.items():
res.append([idx, sum(vals)])
return sorted(res)
Editorial
Approach 1: HashMap
class Solution:
def mergeArrays(
self, nums1: List[List[int]], nums2: List[List[int]]
) -> List[List[int]]:
key_to_sum = {}
# Copying the array nums1 to the map.
for nums in nums1:
key_to_sum[nums[0]] = nums[1]
# Adding the values to existing keys or create new entries.
for nums in nums2:
key_to_sum[nums[0]] = key_to_sum.get(nums[0], 0) + nums[1]
merged_array = []
for key, value in sorted(key_to_sum.items()):
merged_array.append([key, value])
return merged_array
Approach 2: Two Pointers
class Solution:
def mergeArrays(
self, nums1: list[list[int]], nums2: list[list[int]]
) -> list[list[int]]:
N1, N2 = len(nums1), len(nums2)
ptr1, ptr2 = 0, 0
merged_array = []
while ptr1 < N1 and ptr2 < N2:
# If the id is same, add the values and insert to the result.
# Increment both pointers.
if nums1[ptr1][0] == nums2[ptr2][0]:
merged_array.append(
[nums1[ptr1][0], nums1[ptr1][1] + nums2[ptr2][1]]
)
ptr1 += 1
ptr2 += 1
elif nums1[ptr1][0] < nums2[ptr2][0]:
# If the id in nums1 is smaller, add it to result and increment the pointer
merged_array.append(nums1[ptr1])
ptr1 += 1
else:
# If the id in nums2 is smaller, add it to result and increment the pointer
merged_array.append(nums2[ptr2])
ptr2 += 1
# If pairs are remaining in the nums1, then add them to the result.
while ptr1 < N1:
merged_array.append(nums1[ptr1])
ptr1 += 1
# If pairs are remaining in the nums2, then add them to the result.
while ptr2 < N2:
merged_array.append(nums2[ptr2])
ptr2 += 1
return merged_array