Problem Statement
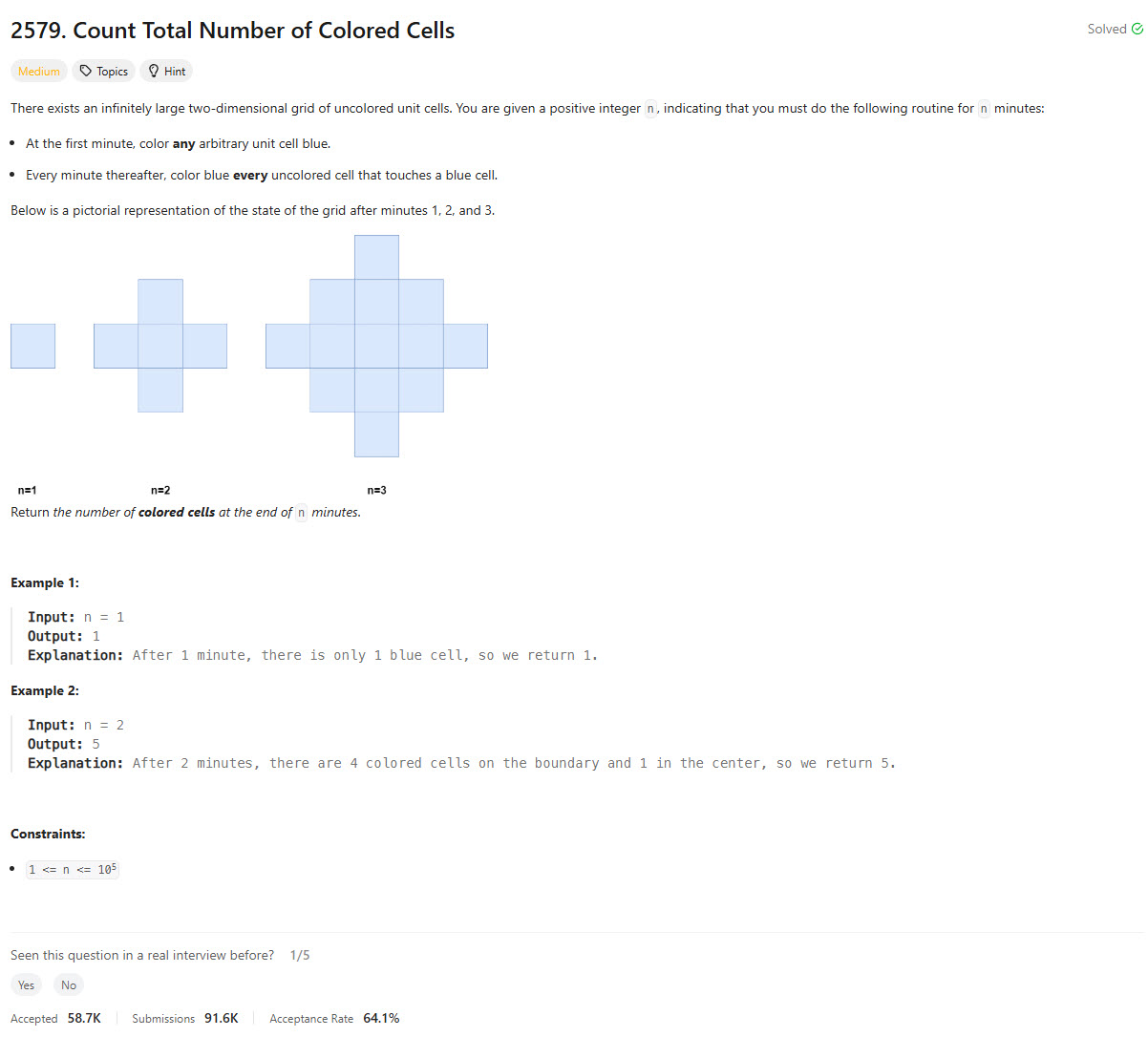
Brute Force - Recursive Approach [Accepted]
class Solution:
def coloredCells(self, n: int) -> int:
def dfs(num, power):
if num == 1:
return 1
return dfs(num - 1, power - 1) + 4*power
return dfs(n, n - 1)
Brute Force - Iterative Approach [Accepted]
class Solution:
def coloredCells(self, n: int) -> int:
i = 1
x = 1
for i in range(1, n + 1):
res = x + 4 * (i - 1)
x = res
return res
Editorial
Approach 1: Iterative Addition
class Solution:
def coloredCells(self, n: int) -> int:
num_blue_cells = 1
addend = 4
# Iterate n - 1 times
while n - 1:
# Add and update current multiple of 4
num_blue_cells += addend
addend += 4
n -= 1
return num_blue_cells
class Solution:
def coloredCells(self, n: int) -> int:
return 1 + n * (n - 1) * 2