Problem Statement
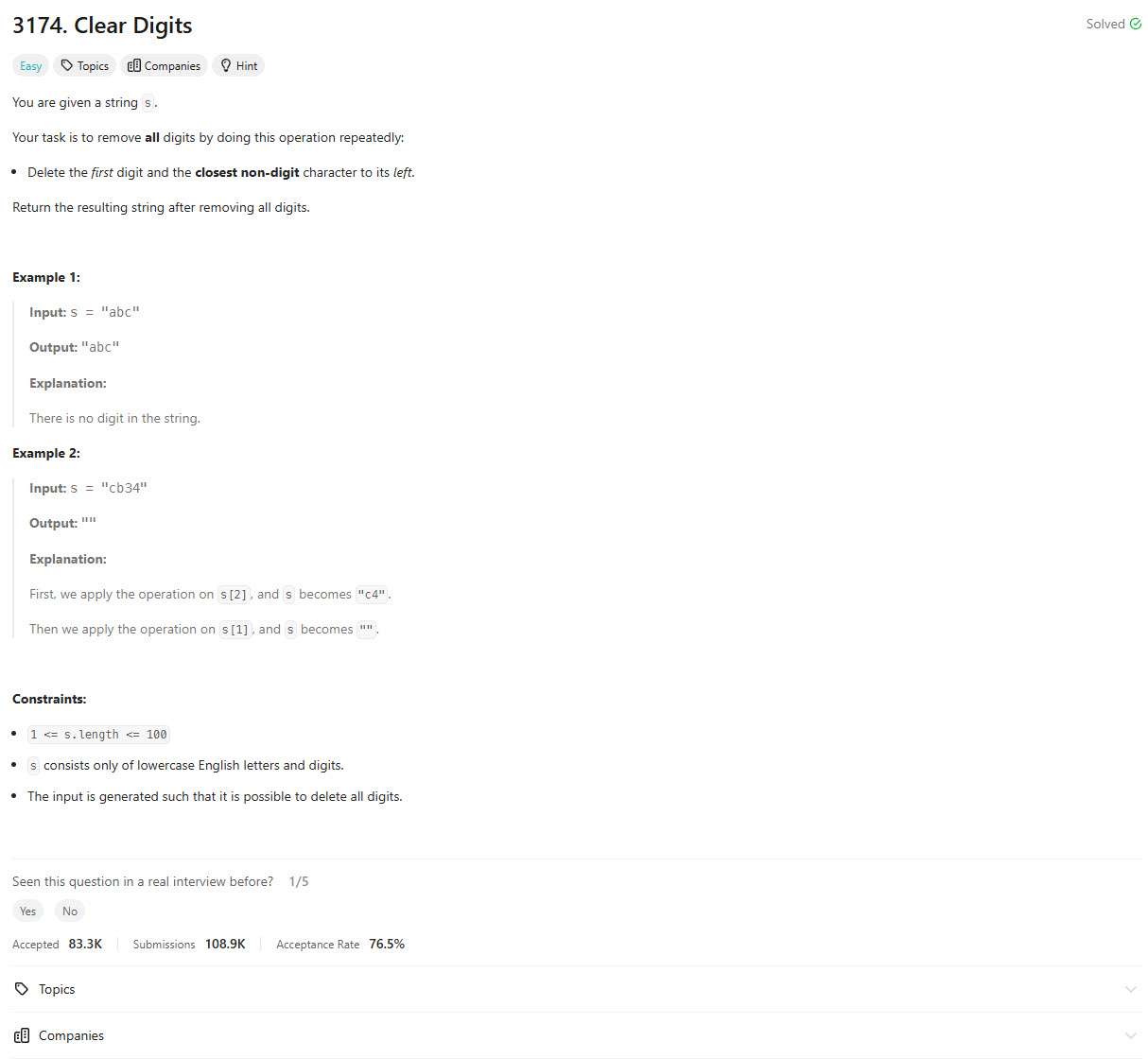
Brute Force [Accepted]
class Solution:
def clearDigits(self, s: str) -> str:
curr = list(s)
while True:
d = deque()
for c in curr:
if c.isalpha():
d.append(c)
else:
d.pop()
curr = list(d)
if len(curr) == len(d):
break
return ''.join(curr)
Editorial
Approach 1: Brute Force
class Solution:
def clearDigits(self, s: str) -> str:
s = list(s)
char_index = 0
# Until we reach the end of the string
while char_index < len(s):
if s[char_index].isdigit():
# Remove the digit from the string
del s[char_index]
# If there is a character to the left of the digit, remove it
if char_index > 0:
del s[char_index - 1]
# Adjust the index to account for the removed character
char_index -= 1
else:
# Move to the next character if it's not a digit
char_index += 1
return "".join(s)
Approach 2: Stack-Like
class Solution:
def clearDigits(self, s: str) -> str:
# Use a list to store characters for efficient modification
answer = []
# Iterate over each character in the input string
for char in s:
# If the current character is a digit
if char.isdigit() and answer:
# If the answer list is not empty, remove the last character
answer.pop()
else:
# If the character is not a digit, add it to the answer list
answer.append(char)
# Join the list back into a string before returning
return "".join(answer)